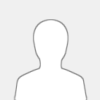 |
These are some notes on using a KC868 as an alarm controller. The functionality is implemented as IFTTT rules implementing the alarm as ladder logic, although I've used text rather than graphical notation because you can't really do ladder logic in ASCII. In this case DI 1-8 are PIR motion sensors and DI 9-12 are smoke alarm sensors. There's sirens/strobes connected to DO 15-16.
In order to be able to do this you need to chain rules which the firmware currently doesn't support but there are requests to add it to the v3 firmware, at the moment I've worked around it by using a relay board to take the wet-contact DO output to the dry-contact DI inputs. When support for this is added to the v3 firmware, e.g. by allowing DO outputs to be tested in IF conditions, then you don't need the external wiring.
- Name = "Smoke", IF { DI 9-12 Rising Edge }, { Sensor1 Temperature >= 50 } THEN { DO On 3 15 16 }
-- If any smoke or heat detector triggers then turn sirens on, chain to rule 8 via DO 3 -> DI 15.
- Name = "Arm", IF { DI 15 Rising Edge } THEN { Delay 5s } { DO On 1 }
-- If arm signal is received then wait 5s and turn on Armed output, chain to rule 7 via DO 1 -> DI 13.
- Name = "Disarm", IF { DI 16 Rising Edge } THEN { DO Off 1 15 16 }
-- If disarm signal is received then turn off Armed output, turn off Siren output, chain to rule 7 via DO 1 -> DI 13.
- Name = "Entrance Motion", IF { DI 1 Rising Edge } THEN { Delay 10s } { DO On 2 }
-- If motion is detected at the entrance then wait 10s and turn on Motion output. This provides an entry delay to disarm the system. Chain to rule 7 via DO 2 -> DI 14.
- Name = "Motion Main", IF { DI 2-4 Rising Edge } THEN { DO On 2 }
-- If motion is detected elsewhere then turn on Motion output, no delay. Chain to rule 7 via DO 2 -> DI 14.
- Name = "Motion Basement", IF { DI 5-8 Rising Edge } THEN { DO On 2 }
-- If motion is detected in the basement then turn on Motion output, no delay. Chain to rule 7 via DO 2 -> DI 14.
Connect DO 1 to DI 13 via NO relay.
Connect DO 2 to DI 14 via NO relay.
- Name = "Alarm Trigger", IF { DI 13 AND DI 14 } THEN { DO On 3 15 16 }
-- If the alarm is armed, DI 13, and motion is detected, DI 14, turn sirens on. Chain to rule 8 via DO 3 -> DI 15.
Connect DO 3 to DI 15 via NO relay.
- Name = "Siren Delay", IF { DI 15-16 } THEN { Delay 300s } { DO Off 1 2 3 15 16 }
-- If sirens are turned on, wait 5 minutes then turn them off again, also turn off all arming signals and triggers.
Rules 2 and 3 handle external arm and disarm signals, e.g. from a keypad by the door, they implement an RS flip-flop to enable triggering in Rule 7. Rule 4 triggers the alarm after a 10s entrance delay to allow disarming. Rules 5 and 6 trigger the alarm immediately. Rule 7 sounds the sirens if the alarm is armed. Rule 8 runs them for 5 minutes, then turns them off again.
There's one bug in this caused by the fact that it's necessary to use an external relay board to chain rules, there's a 5s arming delay but the disarm is immediate, which means if you arm, then disarm, then the arming delay will cause the Arm signal to be sent after the Disarm signal. This needs an extra enabling signal like Rule 7 but I'm out of relay contacts at this point so can't chain in an extra rule. If support is added to the v3 firmware then you can just set another DO and test it in a IF condition so the disarm takes precedence over the arm.
This is a work in progress so there's probably other problems in it, all comments welcome.
|