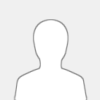 |
Hi all
I can't use relays no turn on and no turn off.
How can you help me?
my code :
#include "gpio_i2c.h"
#include "compile_profile.h"
#include "print_log.h"
#include "PCF8574.h"
#include <Wire.h>
#define MODULE_NAME "gpio_i2c.cpp"
#define Input_IIC_address 0x21
#define Input_IIC_address 0x22
#define Relay_IIC_address 0x24
#define Relay_IIC_address 0x25
GPIO_I2C gpioI2C;
// Set i2c address
PCF8574 pcf8574_input_1(Input_IIC_address,4,5);
PCF8574 pcf8574_input_2(Input_IIC_address,4,5);
PCF8574 pcf8574_relay_1(Relay_IIC_address,4,5);
PCF8574 pcf8574_relay_2(Relay_IIC_address,4,5);
void GPIO_I2C::Init()
{
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
PRINT_LOG_API_PrintDebug("Init()..", MODULE_NAME, __LINE__);
byte count = 0;
Wire.begin(4, 5);
for (byte i = 8; i < 120; i++)
{
Wire.beginTransmission (i); // Begin I2C transmission Address (i)
if (Wire.endTransmission () == 0) // Receive 0 = success (ACK response)
{
Serial.print ("Found address: ");
Serial.print (i, DEC);
Serial.print (" (0x");
Serial.print (i, HEX); // PCF8574 7 bit address
Serial.println (")");
break;
}
}
//Wire.begin(); // Initialize I2C communication
// Wire.setClock(100000); // Set I2C clock to 100kHz (default is 400kHz)
pcf8574_relay_1.pinMode(P0, OUTPUT);
pcf8574_relay_1.pinMode(P1, OUTPUT);
pcf8574_relay_1.pinMode(P2, OUTPUT);
pcf8574_relay_1.pinMode(P3, OUTPUT);
pcf8574_relay_1.pinMode(P4, OUTPUT);
pcf8574_relay_1.pinMode(P5, OUTPUT);
pcf8574_relay_1.pinMode(P6, OUTPUT);
pcf8574_relay_1.pinMode(P7, OUTPUT);
pcf8574_relay_2.pinMode(P0, OUTPUT);
pcf8574_relay_2.pinMode(P1, OUTPUT);
pcf8574_relay_2.pinMode(P2, OUTPUT);
pcf8574_relay_2.pinMode(P3, OUTPUT);
pcf8574_relay_2.pinMode(P4, OUTPUT);
pcf8574_relay_2.pinMode(P5, OUTPUT);
pcf8574_relay_2.pinMode(P6, OUTPUT);
pcf8574_relay_2.pinMode(P7, OUTPUT);
pcf8574_input_1.pinMode(P0, INPUT);
pcf8574_input_1.pinMode(P1, INPUT);
pcf8574_input_1.pinMode(P2, INPUT);
pcf8574_input_1.pinMode(P3, INPUT);
pcf8574_input_1.pinMode(P4, INPUT);
pcf8574_input_1.pinMode(P5, INPUT);
pcf8574_input_1.pinMode(P6, INPUT);
pcf8574_input_1.pinMode(P7, INPUT);
pcf8574_input_2.pinMode(P0, INPUT);
pcf8574_input_2.pinMode(P1, INPUT);
pcf8574_input_2.pinMode(P2, INPUT);
pcf8574_input_2.pinMode(P3, INPUT);
pcf8574_input_2.pinMode(P4, INPUT);
pcf8574_input_2.pinMode(P5, INPUT);
pcf8574_input_2.pinMode(P6, INPUT);
pcf8574_input_2.pinMode(P7, INPUT);
if (pcf8574_relay_1.begin())
{
Serial.println("pcf8574_relay_1 OK");
}
else
{
Serial.println("pcf8574_relay_1 not OK");
}
Serial.print("Init pcf8574_2...");
if (pcf8574_relay_2.begin())
{
Serial.println("pcf8574_relay_2 OK");
}
else
{
Serial.println("pcf8574_relay_2 NOT OK");
}
for(int i=0; i< 8; i++)
{
pcf8574_relay_1.digitalWrite(i, LOW);
pcf8574_relay_2.digitalWrite(i, LOW);
}
if (pcf8574_input_1.begin())
{
Serial.println("pcf8574_input_1 OK");
}
else
{
Serial.println("pcf8574_input_1 not OK");
}
Serial.print("pcf8574_input_2...");
if (pcf8574_input_2.begin())
{
Serial.println("pcf8574_input_2 OK");
}
else
{
Serial.println("pcf8574_input_2 NOT OK");
}
PRINT_LOG_API_PrintDebug("Init()..Finish", MODULE_NAME, __LINE__);
#endif
}
void GPIO_I2C: inMode(uint8_t pinNum, bool mode)
{
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
if (pinNum > 8)
{
if(mode)
{
pcf8574_relay_1.pinMode((pinNum - 1), OUTPUT);
}
}
else
{
if(mode)
{
pcf8574_relay_2.pinMode((pinNum - 1 - 8), OUTPUT);
}
}
#endif
}
void GPIO_I2C::SetPinMode(bool mode, uint8_t pinNum)
{
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
if (pinNum > 8)
{
if(mode)
{
pcf8574_relay_1.pinMode((pinNum - 1), OUTPUT);
}
}
else
{
if(mode)
{
pcf8574_relay_2.pinMode((pinNum - 1 - 8), OUTPUT);
}
}
#endif
}
void GPIO_I2C: igitalWrite(uint8_t pinNum, bool mode)
{
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
if (pinNum > 8)
{
pcf8574_relay_1.digitalWrite(pinNum - 1, mode);
}
else
{
pcf8574_relay_2.digitalWrite(pinNum - 8 - 1, mode);
}
#endif
}
void GPIO_I2C::SetOutputMode(bool mode, uint8_t pinNum)
{
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
if (pinNum > 8)
{
pcf8574_relay_1.digitalWrite(pinNum - 1, mode);
}
else
{
pcf8574_relay_2.digitalWrite(pinNum - 8 - 1, mode);
}
#endif
}
bool GPIO_I2C::ReadInput(uint8_t pinNum)
{
bool retVal = false;
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
if (pinNum > 8)
{
retVal = pcf8574_input_1.digitalRead(pinNum - 1);
}
else
{
retVal = pcf8574_input_2.digitalRead(pinNum - 8 - 1);
}
#endif
return retVal;
}
int GPIO_I2C: igitalRead(uint8_t pinNum)
{
int retVal = -1;
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
if (pinNum > 8)
{
retVal = pcf8574_input_1.digitalRead(pinNum - 1);
}
else
{
retVal = pcf8574_input_2.digitalRead(pinNum - 8 - 1);
}
#endif
return retVal;
}
void GPIO_I2C::TestOutput()
{
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
PRINT_LOG_API_PrintDebug("TestOutput()..", MODULE_NAME, __LINE__);
for(int i=0; i< 8; i++)
{
PRINT_LOG_API_PrintDebug("TestOutput() HIGH", MODULE_NAME, __LINE__);
pcf8574_relay_1.digitalWrite(i, HIGH);
pcf8574_relay_2.digitalWrite(i, HIGH);
delay(2500);
PRINT_LOG_API_PrintDebug("TestOutput() LOW", MODULE_NAME, __LINE__);
pcf8574_relay_1.digitalWrite(i, LOW);
pcf8574_relay_2.digitalWrite(i, LOW);
}
PRINT_LOG_API_PrintDebug("TestOutput()..Finish", MODULE_NAME, __LINE__);
#endif
}
void GPIO_I2C::TestOutputP1()
{
#if ( PROFILE_COMPILE & GPIO_I2C_MODULE)
PRINT_LOG_API_PrintDebug("TestOutputP1() HIGH", MODULE_NAME, __LINE__);
//pcf8574_relay_1.digitalWriteAll(0xff);
//pcf8574_relay_2.digitalWriteAll(0xff);
//pcf8574_1.digitalWrite(0, HIGH);
for (size_t i = 0; i < 8; i++)
{
pcf8574_relay_1.digitalWrite(i, HIGH);
pcf8574_relay_2.digitalWrite(i, HIGH);
}
delay(1000);
PRINT_LOG_API_PrintDebug("TestOutputP2() LOW", MODULE_NAME, __LINE__);
for (size_t i = 0; i < 8; i++)
{
pcf8574_relay_1.digitalWrite(i, LOW);
pcf8574_relay_2.digitalWrite(i, LOW);
}
delay(1000);
#endif
}
|