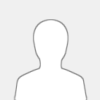 |
[b]1.Diagram of relay Controller[/b]
![[Image: KC868-Server2_02.png]](https://www.kincony.com/images/kc868-server/KC868-Server2_02.png)
Download PDF details
[b]Leds status different color means:[/b]
Red: Controller is power on.
Green: WiFi module is connect to your router successfully.
White: network run in “TCP Client” mode or “MQTT” mode.
Yellow: network run in “TCP Server” mode.
Blue: network run in “UDP” mode.
[b]what’s UDP , TCP Server, TCP Client, MQTT means:[/b]
When set to “UDP” and set the cloud server IP and port, controller will auto connect to KinCony’s Cloud server. Then you can use “KinCony Smart Home” phone app for remote control relay.
When set to “TCP Server”, controller own is a server work in local network ,you can use “KBOX” phone app without internet and our cloud server to control relay in local network.just your mobile phone or PC and controller connect with same router.
When set to “TCP Client” and set the server IP and port, controller will auto connect to your own server (not KinCony’s cloud server) by “TCP Client”, you can write program for server and make your own phone app to use, this for developer to use.
When set to “MQTT” and set the server IP and port, controller will auto connect to KinCony’s MQTT cloud server or your own server (not KinCony’s cloud server) by MQTT, you can write program for server and make your own phone app to use, this for developer to use. Or you can use “KBOX” android phone app for remote control.
[b]what’s buttons function:[/b]
Button1 “WiFi Reset” : Reset WiFi module, then controller will be work as “AP” mode, you will see the wifi signal “LPT230” for use.Now you can config the ssid and password for your router.
Button2 “Ethernet Mode” : change ethernet work mode for UDP->TCP Server->TCP Client.
Button3 “WiFi Mode” : change WiFi work mode for UDP->TCP Server->TCP Client.
[b]How to use buttons:[/b]
[b]Button1 “WiFi Reset” :[/b] Hold on the button for 5 seconds,then restart power of controller.
[b]Button2 “Ethernet Mode” :[/b]
Hold on the button until LED color changed. There are 5 status:
a.) Hold on button, when the [yellow LED] is ON, release it, then work mode in “TCP server” mode;
b.) Hold on button, when the [white LED] is ON, release it, then work mode in “TCP Client” mode;
c.) Hold on button, when the [blue LED] is ON, release it, then work mode in “UDP” mode;
d.) Hold on button, when the [yellow and white LED] all is ON, release it, then work mode in “UDP” and IP by DHCP mode;
e.) Hold on button, when the [yellow and white and blue LED] all is ON, release it, then work mode in “TCP Server” and IP (192.168.1.200) by static mode;
Note: after changed mode, the controller will restart automatically.
The controller default work mode is DHCP and UDP mode.
[b]Button3 “WiFi Mode” :[/b]
Hold on the button until LED color changed. There are 3 status:
a.) Hold on button, when the [yellow LED] is ON, release it, then work mode in “TCP server” mode;
b.) Hold on button, when the [white LED] is ON, release it, then work mode in “TCP Client” mode;
c.) Hold on button, when the [blue LED] is ON, release it, then work mode in “UDP” mode;
Note: after changed mode, the controller will restart automatically.
The controller default work mode is DHCP and UDP mode.
[b]2.Network Setting[/b]
A.WiFi config (if you use ethernet cable, you can skip WiFi config step):
B.Set Work Mode for remote control (Need internet and KinCony’s cloud server)
C.Set Work Mode for local LAN control (without internet)
[b]A-1.use Ethernet port to config IP and different work mode by “KinCony-SCAN_Device Tool” for KC868-xB/xBS” series (Recommend)[/b]
Let your KinCony smart controller connect to your router by ethernet cable (CAT5) and your computer also connect with your same router, download “KinCony-SCAN_Device Tool” and unzip file, open “KinCony-SCAN_Device.exe”
![[Image: ip-scan-1.png]](https://www.kincony.com/images/user-guide/ip-scan/ip-scan-1.png)
![[Image: kc868-server.png]](https://www.kincony.com/images/user-guide/kc868-server/kc868-server.png)
Step1: chose your network device of your computer.
Step2: click “StartMonitorPort” button.
Step3: click “SCAN” button.
Now all KinCony Controller (KC868-HxB or KC868-HxBS) will be see. You will see the device IP,Port,UID,type.
![[Image: kc868-server-login.png]](https://www.kincony.com/images/user-guide/kc868-server/kc868-server-login.png)
web browser login with your KC868-Server’s IP Address, user and password default all are : admin admin
![[Image: kc868-server-control-panel.png]](https://www.kincony.com/images/user-guide/kc868-server/kc868-server-control-panel.png)
Here is “Relay Control” panel webpage, you can control every channel output independently.
![[Image: Network-Setting-size-small.png]](https://www.kincony.com/images/user-guide/kc868-server/Network-Setting-size-small.png)
Here is network setting details.
[b]SSID and password:[/b] These are your router’s.
[b]Work Mode:[/b]
1- UDP: Connect with KinCony Cloud server by internet, you can use “KinCony Smart Home ” mobile phone application.
2- TCP Server: Work in local network without internet, you can use “KBOX” mobile phone application.
3- TCP Client: This mode for programmer or developer, controller can connect to your own cloud server by TCP socket connection.
4- MQTT: Connect to any MQTT broker by IP and Port.
[b]Domain name:[/b] connnect to cloud server by domain name, KC868-Server will use DNS to find IP address for cloud server
[b]Post Password:[/b] for http command line use
[b]SW trigger output:[/b] 16 channel output action link or unlink with 16 channel input
[b]Input filter time:[/b] Valid holding time can trigger the input for Digital Input 1-8 ports
[b]B.WiFi advanced config by hotspot without router: (Most time not need to use, you can skip this step)[/b]
1. Install WiFi antenna for Controller.
2. Make sure “DHCP” function is enabled by your router.
3. Enable your computer WiFi network.
4. Begin to config WiFi as follow steps:
Let your computer to connect WiFi wireless network name of “LPT230”
![[Image: wifi-ap-1.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-ap-1.jpg)
When wifi connected, open browser visit: http://10.10.100.254/
![[Image: wifi-config-1.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-1.jpg)
![[Image: wifi-config-2.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-2.jpg)
input the user name: admin password: admin
![[Image: wifi-config-3.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-3.jpg)
now the default menu is Chinese , you can click “English” change to English language menu.
![[Image: wifi-config-4.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-4.jpg)
this is English menu.
![[Image: wifi-config-5.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-5.jpg)
Enter wifi work mode, default is “AP mode”
![[Image: wifi-config-6.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-6.jpg)
Now we change to “STA mode”, “STA” means “Station”
![[Image: wifi-config-7.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-7.jpg)
not need to “Restart” , just click “Back” button for other settings, at last you need Restart once.
![[Image: wifi-config-8.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-8.jpg)
Press “Scan” to search your router’s wifi signal or you input manually.
![[Image: wifi-config-9.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-9.jpg)
Chose your router’s wifi ssid
![[Image: wifi-config-10.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-10.jpg)
there is a tip message for you to input password of your router.
![[Image: wifi-config-11.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-11.jpg)
now input password of your router.
![[Image: wifi-config-12.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-12.jpg)
also press “Back” begin for other settings.
[b]Note:[/b]
If you want to reset wifi for new config, you can use WiFi Reset button for re-config.
[b]B.Set Work Mode for remote control (Need internet and KinCony’s cloud server)[/b]
![[Image: wifi-config-13.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-13.jpg)
now you want to use “KinCony smart home ” phone app for remote control, so make sure your Protocol line is “UDP” and Server address is “114.55.89.143”,Port is “5555”. now all setting is finished, we press “Save”, then restart.
![[Image: wifi-config-14.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-14.jpg)
now you can restart power of your controller. Now you can use “KinCony Smart Home” app and PC software for remote control.
[b]C.Set Work Mode for local LAN control (without internet)[/b]
![[Image: wifi-config-15.jpg]](https://www.kincony.com/images/kc868-h32b/user-guide/wifi-config-15.jpg)
Just change Protocol = TCP Server after “Save” Re-start power for controller. Now you can use “KBOX Smart” app and PC software for local control.
[b]3. How to use Phone APP and PC software in WAN/LAN[/b]
[b]Click below photo to see video:[/b]
A.APP- KinCony Smart Home (WAN:need internet)
![[Image: kincony-smart-home.JPG]](https://www.kincony.com/images/user-guide/app-photo/kincony-smart-home.JPG)
B.APP- KBOX Smart (LAN:without internet)
![[Image: kbox.png]](https://www.kincony.com/images/user-guide/app-photo/kbox.png)
C.PC Software (LAN:without internet)
![[Image: smart-controller-pc-software.jpg]](https://www.kincony.com/images/user-guide/app-photo/smart-controller-pc-software.jpg)
D.PC Software (WAN:need internet)
|